Mastering Dependency Injection in C#: Boost Your Code's Flexibility and Testability
Dive deep into Dependency Injection in C# and discover how it can transform your programming approach, leading to more maintainable and testable code.
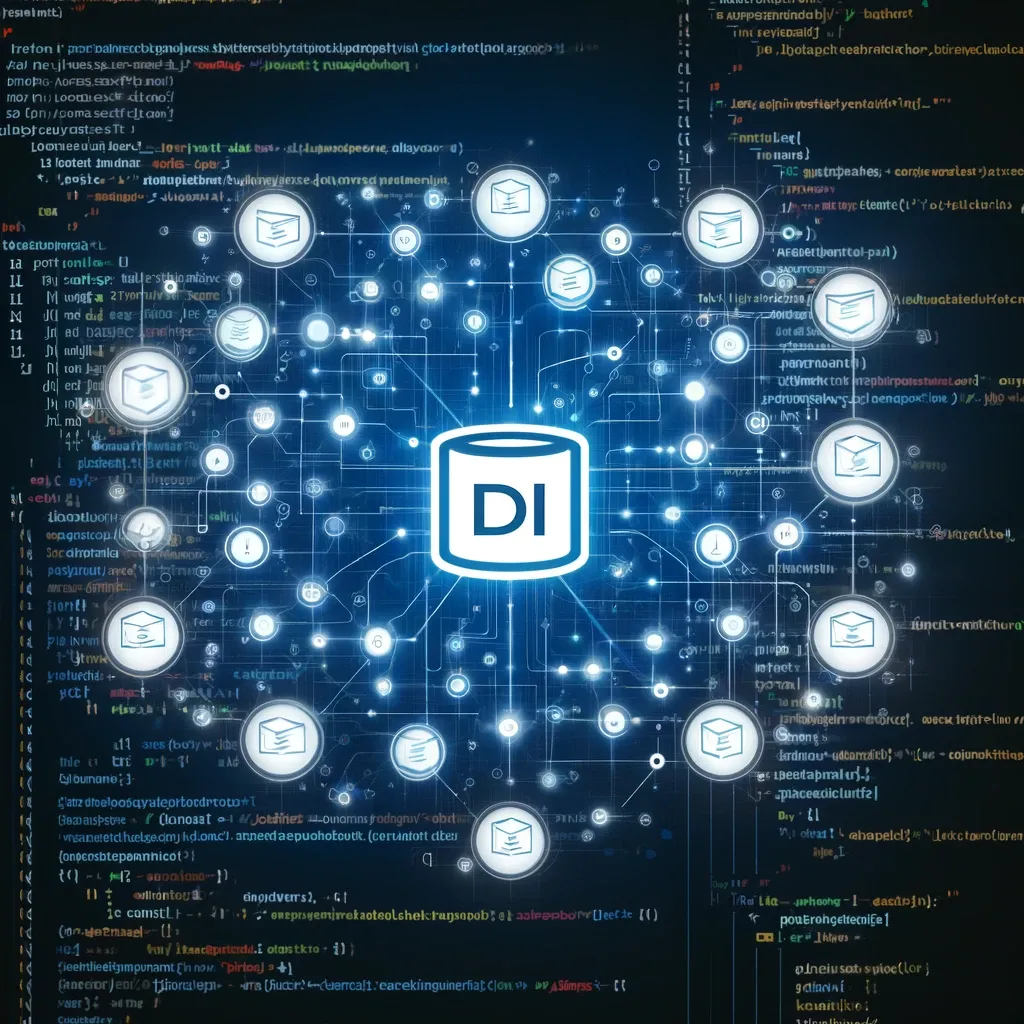
Unlock the full potential of your C# applications with our comprehensive guide on Dependency Injection. Learn how DI can enhance your code's flexibility, maintainability, and testability.
Dependency Injection in C#: A Key to More Maintainable Code
In the bustling world of software development, writing clean, maintainable, and testable code is the holy grail. Enter Dependency Injection (DI) - a design pattern that's as much about managing your application's dependencies as it is about elevating your coding game. This guide is your ticket to understanding and implementing DI in C#, ensuring your projects are not just good, but great.
Why Bother with Dependency Injection?
At its core, DI is all about decoupling. It's the process of providing the objects that an object needs (its dependencies) instead of having it construct them itself. It's a subtle shift in mindset but one that pays dividends in code maintainability, scalability, and testability. Here’s why:
- Reduced Coupling: By decoupling objects from their dependencies, you make your code more resilient to changes. If you need to switch out a database or a third-party service, DI makes it a breeze.
- Increased Flexibility: With DI, you can easily swap out implementations for testing, development, or production environments.
- Enhanced Testability: DI allows for easier unit testing since you can inject mock dependencies, making your tests cleaner and more reliable.
The Nuts and Bolts of Dependency Injection in C#
So, how does one implement DI in C#? At its simplest, DI can be done manually, but more complex applications might benefit from using a DI container, such as .NET's built-in Microsoft.Extensions.DependencyInjection
.
Let’s break it down with an example. Imagine you have a UserService
class that requires an IUserRepository
to access user data. Without DI, you might instantiate a UserRepository
directly within your UserService
. With DI, UserService
simply declares an IUserRepository
in its constructor, and it's the job of the DI framework to supply an instance at runtime.
public class UserService
{
private readonly IUserRepository _userRepository;
public UserService(IUserRepository userRepository)
{
_userRepository = userRepository;
}
// Methods using _userRepository to perform actions...
}
Implementing DI with .NET Core's Built-in Container
.NET Core (and .NET 5+) comes with a powerful, yet straightforward DI container that you can use to register and resolve dependencies. Here's a quick how-to:
- Register Dependencies: In the
Startup.cs
of your application, you use theConfigureServices
method to add your services and their dependencies.
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped<IUserRepository, UserRepository>();
services.AddScoped<UserService>();
}
- Injecting Dependencies: Once registered, .NET's DI container will automatically inject these dependencies wherever needed.
public class SomeController : ControllerBase
{
private readonly UserService _userService;
public SomeController(UserService userService)
{
_userService = userService;
}
// Use _userService in your controller actions...
}
Best Practices for Dependency Injection in C#
While DI can seem like a silver bullet, it's essential to use it judiciously. Here are some best practices to keep in mind:
- Prefer Interfaces to Concrete Classes: This makes your code more modular and testable.
- Avoid Service Locator Pattern: It hides class dependencies, making your code harder to understand and maintain.
- Be Mindful of the Lifetime of Dependencies: .NET Core's DI container offers different lifetimes (singleton, scoped, transient) for your services. Choose wisely based on your use case.
Wrapping Up
Dependency Injection isn't just a fancy technique; it's a foundational principle for modern software development in C#. By decoupling your classes from their dependencies, you make your code more flexible, maintainable, and, importantly, testable. Whether you're a seasoned developer or just starting out, embracing DI will undoubtedly elevate your C# projects to the next level.
FAQs About Dependency Injection in C#
- What is the best DI container for C#?
While .NET's built-in DI container is powerful and suitable for most needs, other options like Autofac and Unity offer additional features and flexibility. - Can I use DI in .NET Framework applications?
Yes, while .NET Core and .NET 5+ have built-in support for DI, you can use third-party libraries like Autofac in .NET Framework applications to achieve similar functionality. - Is it okay to have multiple constructors in a DI-enabled class?
While technically possible, having multiple constructors can complicate the resolution of dependencies. It's generally best to have a single constructor that defines all required dependencies.
Embarking on your Dependency Injection journey in C# can truly transform how you approach software development. It encourages better design practices, makes your codebase more adaptable, and significantly eases the testing process. So, why not give it a shot and see how it can elevate your projects? Your code (and your future self) will thank you.